Unified Cloud Infrastructure
CDN
Cloud Server
Object Storage
Video Platform
Cloud Container
DBaaS
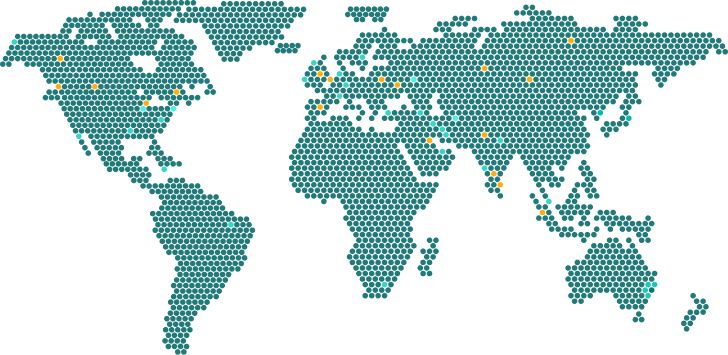
Apply your favorite strategy based on your business scale and industry. It’s easy and straightforward to use ArvanCloud’s products, yet if you have any difficulties with service implementation, feel free to contact our team of experts. We are here to guide you around the clock for an incredibly safe, fast, and effortless migration.